Introduction to Strings in Java
- Vaishnavi
- Sep 24, 2022
- 2 min read
Updated: Sep 25, 2022
What are Strings?
Strings are used to store a sequence of characters. For example, the name of a person, designation of an employee, name of a fruit, etc.
What are Strings in Java?
The String is a Reference data type that is used to store a sequence of characters.
The String is a class in java.lang package.
String API contains many built-in operations like compare, concat, equals, split, length, compareTo, substring, etc.
String objects are stored in the Heap memory area.
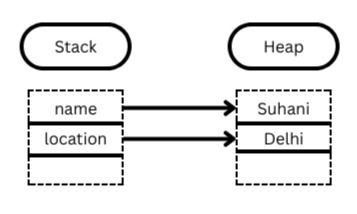
** The reference variables are stored in the stack memory area.
Creation of String
The string can be created in Java in 2 ways:-
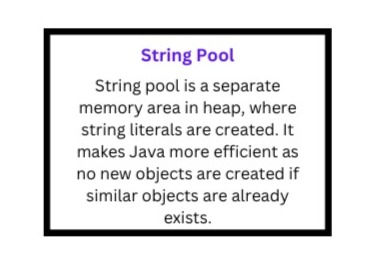
Created in String Pool
String name = "Suhani"
String location = "Delhi"
String name1 = "Suhani"
Created By a new keyword
String name2 = new String("Alia");
String name3 = new String("Alia");
In the first case, the string gets created in the String pool and in the second case, a new object is created.
Strings are Immutable In java
Immutable means something that cannot be modified or changed once created.
Strings are immutable in Java. Once a String object is created its data or state can't be changed but a new string object is created.
For Example:- String name = "Mini"
name = "Sona"
Here, the string object "Mini" can't be modified to "Sona". Instead, a new object "Sona" is created and mapped with the name reference variable.
String name="Mini";
String name1="Mini";
String name2=new String("Mini");
System.out.println(name == name1); --------------> true
System.out.println(name == name2); --------------> false
System.out.println(name.equals(name2)); --------------> true
Built-In Methods of String API

length() - Returns the length of the string
concat() - Combines the given string object with the called object.
charAt() - Returns the character at a given index [0 - length-1].
equals() - Checks for the values inside the object to be compared.
substring() - Returns a new string object containing the substring of a given string.
String Buffer
As strings are immutable in java. To create a mutable string in java we can use a String Buffer.
It does not use the string pool concept. All the Objects of String Buffer are thread-safe.
StringBuffer sb = new StringBuffer("Java");
StringBuffer Functions
append() - Appends the specified string with the given string, hence, the original string gets modified.
length()
charAt()
substring()
reverse()
StringBuffer to String
StringBuffer objects can be converted to String so that we can manipulate String functions on them. This can be done using the method toString(). The toString method is present in Object Class and returns the String representation of the reference Object.
For example, To check whether the given StringBuffer Object’s value is equal to Raghu, we can convert StringBuffer to the String and use the equals method on it.
StringBuffer name= new StringBuffer("Raghu");
String sname=name.toString();
System.out.println(sname.equals("Raghu"));
created by Vaishnavi Chaurasia @TechInfinity
Comments